Essentially the same procedure, from a development perspective, as in 10g -
but under the hood we're using the 11g Infrastructure layer.
The Oracle documentation can be found at
http://download.oracle.com/docs/cd/E12839_01/integration.1111/e10224/bp_java.htm#BABCBEJJ
Here's a simple example based on WSDL Java binding, later posts will cover bpel:exec etc. -
1. Create a generic Application/Project in Jdev 11g
1.1. Add 2 classes - Cust & Greeting
Cust -
package cccwsif;
public class Cust {
private String custName1;
private String custName2;
private String custXMASgreeting;
public Cust() {
super();
}
public void setCustName1(String custName1) {
this.custName1 = custName1;
}
public String getCustName1() {
return custName1;
}
public void setCustName2(String custName2) {
this.custName2 = custName2;
}
public String getCustName2() {
return custName2;
}
public void setCustXMASgreeting(String custXMASgreeting) {
this.custXMASgreeting = custXMASgreeting;
}
public String getCustXMASgreeting() {
return custXMASgreeting;
}
}
Greeting -
package cccwsif;
public class Greeting {
public Greeting() {
super();
}
public Cust XMASgreet(Cust c){
String g = "Happy Christmas " + c.getCustName1() + " " +
c.getCustName2();
c.setCustXMASgreeting(g);
return c;
}
}
1.2. expose Greeting as a Web Service
1.2.1. Right-mouse click on Greeting
1.2.2. Then select "Create Web Service..."
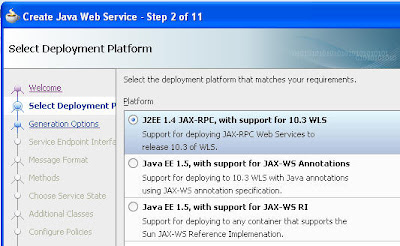
1.2.3. Accept defaults for all steps up until step 9.
1.2.4. Additional Classes --> Include "Cust" class
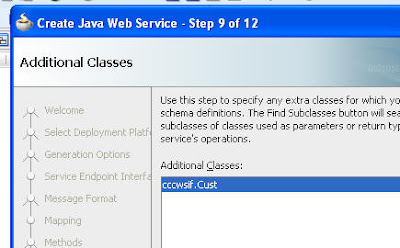
1.2.5. open the wsdl file and set nillable=false
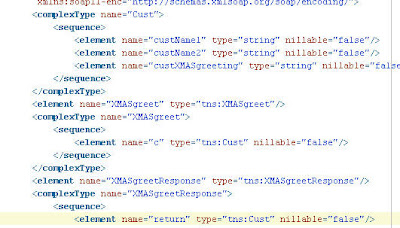
1.2.6. open the GreetingService-java-wsdl-mapping.xml file
1.2.7. check the mapping order
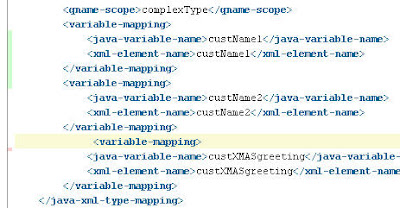
1.3. Add the Java Binding to the WSDL
1.3.1. Open the wsdl in "Design" mode
1.3.2. Click the + by Bindings
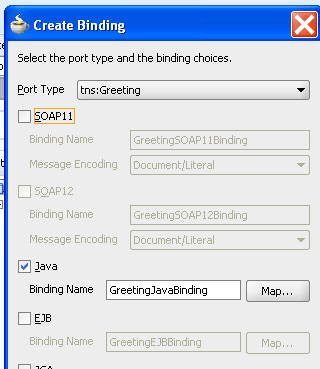
1.3.3. Click the Map button
1.3.4. select the Cust class
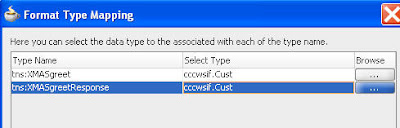
1.3.5. Amend the Service entry in the WSDL as follows -
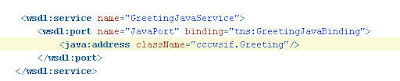
1.4.1. File --> New --> Deployment Profile --> Jar
1.4.2. Deploy to Jar
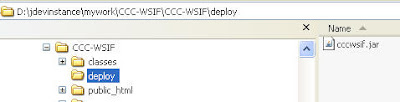
2. Create a SOA App in JDev 11g
2.1. Copy the WSDL into the project
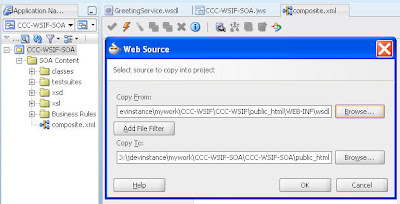
2.2. Drop a BPEL service component onto the designer
2.2.1. Set input / output as follows -
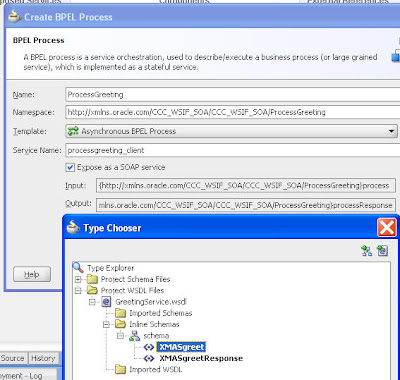
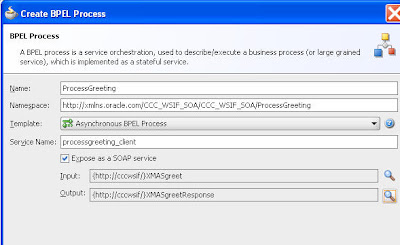
2.3. Add a partner Link to the process, pointing to the imported WSDL
2.3.1. Add Assign - Invoke - Assign Activities
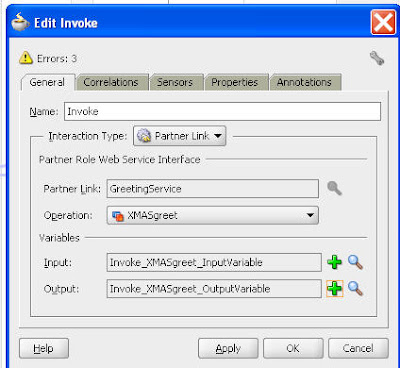
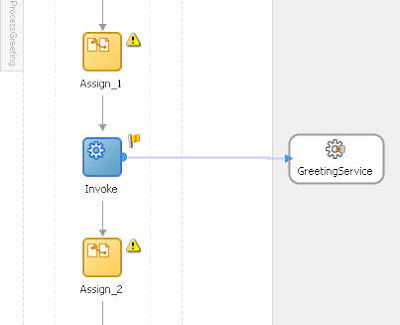
2.3.2 Assign input / output vars in the 2 Assigns
2.4. Copy the Jar file from the previous project to the SOA Project sca-inf\lib directory
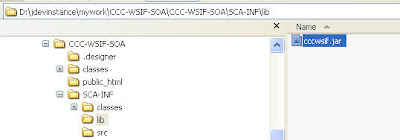
2.5. Deploy the SOA App
3 Test
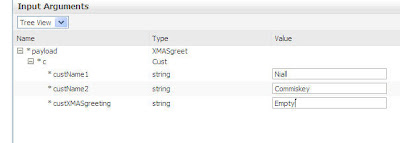
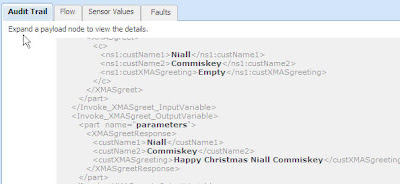